Flutter, developed by Google, is a popular open-source UI toolkit for building natively compiled applications for mobile, web, and desktop from a single codebase. As a beginner, mastering Flutter can seem daunting, but with the right tips and tricks, you can enhance your productivity and create stunning applications. This guide provides ten essential Flutter tips and tricks for beginners to help you get started on the right foot.
1. Set Up Your Development Environment
Install Flutter and Dart
Before diving into Flutter development, ensure you have the Flutter SDK and Dart installed on your system. Follow the official Flutter installation guide to set up your development environment.
Use an IDE with Flutter Support
Choose an Integrated Development Environment (IDE) that supports Flutter, such as Android Studio or Visual Studio Code. These IDEs offer excellent tools and plugins for Flutter development, including code completion, debugging, and widget inspection.
2. Understand the Widget Tree
The Widget Concept
In Flutter, everything is a widget. Widgets are the building blocks of a Flutter application, representing both structural elements (such as buttons and text) and stylistic elements (such as padding and margins).
Building a Widget Tree
A Flutter application is essentially a tree of widgets. Understanding the widget tree and how to nest widgets properly is crucial for creating complex and responsive UIs.
import 'package:flutter/material.dart';
void main() => runApp(MyApp());
class MyApp extends StatelessWidget {
@override
Widget build(BuildContext context) {
return MaterialApp(
home: Scaffold(
appBar: AppBar(title: Text('Flutter Tips')),
body: Center(child: Text('Hello, Flutter!')),
),
);
}
}
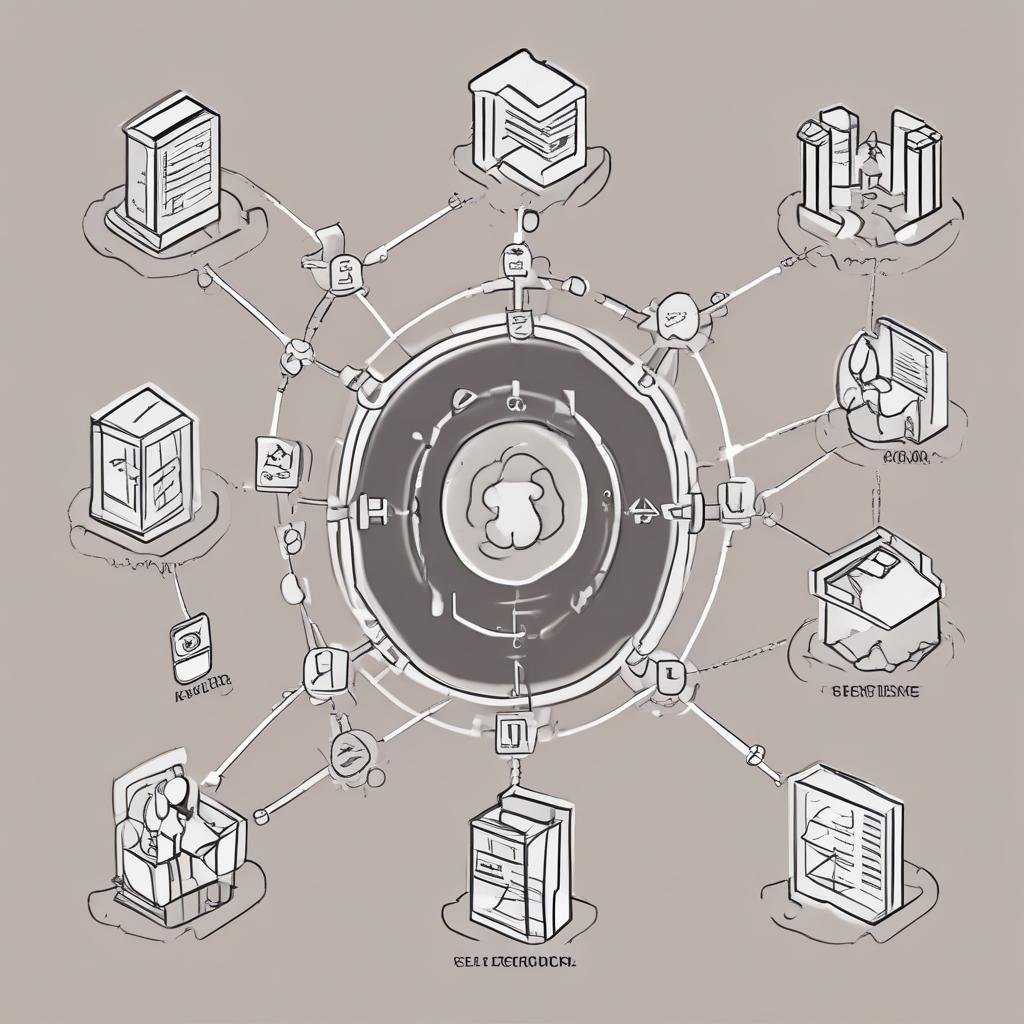
3. Use Hot Reload for Rapid Development
What is Hot Reload?
Hot reload is one of Flutter’s most powerful features, allowing you to see the results of code changes almost instantly without restarting your app. This speeds up the development process and enables quick experimentation.
How to Use Hot Reload
To use hot reload, simply save your code changes, and Flutter will automatically update the running app. This feature is available in both Android Studio and Visual Studio Code.
4. Leverage Flutter’s Rich Widget Library
Commonly Used Widgets
Flutter comes with a rich library of pre-built widgets that you can use to build your application. Some commonly used widgets include:
- Container: A versatile widget for layout and styling.
- Row and Column: For arranging widgets horizontally and vertically.
- ListView: For displaying scrollable lists of widgets.
- Stack: For overlapping widgets.
Customizing Widgets
You can customize widgets by modifying their properties or combining them with other widgets. This flexibility allows you to create unique and tailored user interfaces.
Container(
padding: EdgeInsets.all(16.0),
margin: EdgeInsets.all(8.0),
decoration: BoxDecoration(
color: Colors.blue,
borderRadius: BorderRadius.circular(8.0),
),
child: Text('Customized Container'),
)
5. Manage State Effectively
Stateful vs. Stateless Widgets
Flutter provides two types of widgets for managing state: StatelessWidget and StatefulWidget. Use StatelessWidget for static content and StatefulWidget for dynamic content that can change during runtime.
Using setState for State Management
To update the state of a StatefulWidget, use the setState
method. This method triggers a rebuild of the widget with the new state.
class MyStatefulWidget extends StatefulWidget {
@override
_MyStatefulWidgetState createState() => _MyStatefulWidgetState();
}
class _MyStatefulWidgetState extends State<MyStatefulWidget> {
int _counter = 0;
void _incrementCounter() {
setState(() {
_counter++;
});
}
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(title: Text('State Management')),
body: Center(
child: Column(
mainAxisAlignment: MainAxisAlignment.center,
children: [
Text('Counter: $_counter'),
ElevatedButton(
onPressed: _incrementCounter,
child: Text('Increment'),
),
],
),
),
);
}
}
6. Use Navigator for Routing
Navigating Between Screens
Flutter’s Navigator widget allows you to manage your app’s routes and navigate between screens. Use Navigator.push
to navigate to a new screen and Navigator.pop
to return to the previous screen.
Navigator.push(
context,
MaterialPageRoute(builder: (context) => SecondScreen()),
);
Passing Data Between Screens
You can pass data between screens by including it in the MaterialPageRoute
constructor and accessing it in the new screen’s constructor.
class FirstScreen extends StatelessWidget {
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(title: Text('First Screen')),
body: Center(
child: ElevatedButton(
onPressed: () {
Navigator.push(
context,
MaterialPageRoute(
builder: (context) => SecondScreen(data: 'Hello from First Screen'),
),
);
},
child: Text('Go to Second Screen'),
),
),
);
}
}
class SecondScreen extends StatelessWidget {
final String data;
SecondScreen({required this.data});
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(title: Text('Second Screen')),
body: Center(child: Text(data)),
);
}
}
7. Use Themes for Consistent Styling
Defining a Theme
Themes allow you to define and apply consistent styling throughout your Flutter application. Use the ThemeData
class to define your theme and apply it to the MaterialApp
widget.
MaterialApp(
theme: ThemeData(
primaryColor: Colors.blue,
accentColor: Colors.orange,
textTheme: TextTheme(
bodyText2: TextStyle(color: Colors.black),
),
),
home: MyHomePage(),
);
Customizing Widgets with Themes
You can customize widgets by defining theme properties and using the Theme.of
method to access the theme in your widgets.
Container(
color: Theme.of(context).primaryColor,
child: Text(
'Themed Container',
style: Theme.of(context).textTheme.bodyText2,
),
)
8. Use Provider for State Management
What is Provider?
Provider is a popular state management library in Flutter that makes it easy to manage and propagate state across your application. It uses the InheritedWidget under the hood and provides a simple API for state management.
Using Provider
To use Provider, add the provider
package to your pubspec.yaml
file and define a provider in your widget tree.
import 'package:provider/provider.dart';
void main() => runApp(
ChangeNotifierProvider(
create: (context) => Counter(),
child: MyApp(),
),
);
class Counter with ChangeNotifier {
int _count = 0;
int get count => _count;
void increment() {
_count++;
notifyListeners();
}
}
Consuming the Provider
Use the Consumer
widget or Provider.of
method to access the provider’s state and trigger updates when the state changes.
class MyHomePage extends StatelessWidget {
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(title: Text('Provider Example')),
body: Center(
child: Consumer<Counter>(
builder: (context, counter, child) {
return Text('Counter: ${counter.count}');
},
),
),
floatingActionButton: FloatingActionButton(
onPressed: () => Provider.of<Counter>(context, listen: false).increment(),
child: Icon(Icons.add),
),
);
}
}
9. Optimize Performance
Use Const Constructors
Use const
constructors for widgets that do not change during runtime. This helps Flutter optimize the widget tree and improve performance.
const Text('Hello, Flutter!');
Avoid Rebuilding Widgets Unnecessarily
Minimize the number of widget rebuilds by using efficient state management and avoiding unnecessary updates to the widget tree.
Use the Flutter DevTools
Flutter DevTools provides a suite of performance and debugging tools to help you optimize your application. Use the DevTools to profile your app, identify performance bottlenecks, and analyze widget rebuilds.
10. Explore Flutter’s Ecosystem
Flutter Packages
Leverage the extensive Flutter ecosystem by using packages from the pub.dev repository. These packages can save you time and effort by providing pre-built functionality for common tasks.
Community Resources
Join the Flutter community to stay updated with the latest developments and best practices. Participate in forums, attend meetups, and follow official Flutter channels for tutorials and updates.
Conclusion
Mastering Flutter as a beginner involves understanding the basics, leveraging powerful features, and following best practices. By implementing these ten essential tips and tricks, you’ll be well on your way to creating impressive and efficient