Python’s rich ecosystem of libraries is one of the reasons for its widespread popularity among developers. Libraries extend the capabilities of Python, enabling developers to perform tasks ranging from data manipulation to web development with ease. In this article, we’ll explore some of the most essential Python libraries that every developer should be familiar with.
1. NumPy
NumPy (Numerical Python) is a fundamental library for numerical computing in Python. It provides support for arrays, matrices, and a plethora of mathematical functions to operate on these data structures.
- Key Features:
- High-performance array objects
- Mathematical functions for array operations
- Linear algebra functions
- Random number generation
Example Usage:
import numpy as np
# Creating a NumPy array
array = np.array([1, 2, 3, 4])
print(array.mean()) # Output: 2.5
2. Pandas
Pandas is a powerful library for data manipulation and analysis. It provides data structures like Series and DataFrames that are essential for handling structured data.
- Key Features:
- DataFrames and Series for data manipulation
- Tools for reading and writing data in various formats (CSV, Excel, SQL, etc.)
- Data cleaning and preprocessing capabilities
Example Usage:
import pandas as pd
# Creating a DataFrame
data = {'Name': ['Alice', 'Bob'], 'Age': [30, 25]}
df = pd.DataFrame(data)
print(df)
3. Matplotlib
Matplotlib is a plotting library that enables the creation of static, animated, and interactive visualizations in Python. It is widely used for data visualization.
- Key Features:
- Customizable plots
- Support for various plot types (line plots, scatter plots, histograms, etc.)
- Integration with other libraries like NumPy and Pandas
Example Usage:
import matplotlib.pyplot as plt
# Creating a simple line plot
plt.plot([1, 2, 3, 4], [10, 20, 25, 30])
plt.xlabel('X-axis')
plt.ylabel('Y-axis')
plt.title('Sample Plot')
plt.show()
4. Seaborn
Seaborn is a statistical data visualization library based on Matplotlib. It provides a high-level interface for drawing attractive and informative statistical graphics.
- Key Features:
- Advanced data visualization tools
- Integration with Pandas DataFrames
- Built-in themes for styling plots
Example Usage:
import seaborn as sns
import matplotlib.pyplot as plt
# Creating a bar plot with Seaborn
data = {'Category': ['A', 'B', 'C'], 'Value': [10, 20, 30]}
df = pd.DataFrame(data)
sns.barplot(x='Category', y='Value', data=df)
plt.show()
5. Scikit-learn
Scikit-learn is a comprehensive library for machine learning in Python. It provides simple and efficient tools for data mining and data analysis.
- Key Features:
- A wide range of machine learning algorithms
- Tools for model selection and evaluation
- Support for data preprocessing
Example Usage:
from sklearn.linear_model import LinearRegression
# Creating a linear regression model
model = LinearRegression()
X = [[1], [2], [3], [4]]
y = [10, 20, 25, 30]
model.fit(X, y)
print(model.predict([[5]])) # Output: [35.]
6. Flask
Flask is a lightweight web framework for Python. It’s designed to be simple and easy to extend, making it ideal for building web applications.
- Key Features:
- Minimalist design for creating web apps
- Extensible with a variety of plugins
- Built-in development server
Example Usage:
from flask import Flask
app = Flask(__name__)
@app.route('/')
def home():
return "Hello, Flask!"
if __name__ == '__main__':
app.run(debug=True)
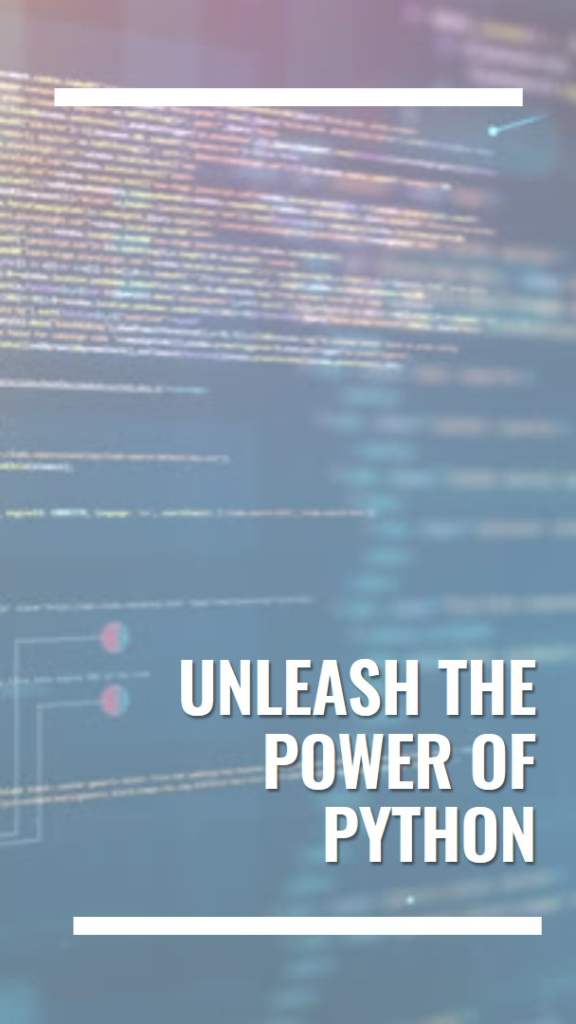
7. Django
Django is a high-level web framework that encourages rapid development and clean, pragmatic design. It includes everything needed to build a web application.
- Key Features:
- Built-in admin interface
- ORM (Object-Relational Mapping) for database interactions
- Security features and authentication
Example Usage:
# Starting a new Django project (from command line)
django-admin startproject myproject
Conclusion
ecosystem significantly enhances its functionality, making it an ideal choice for a wide range of applications. From numerical computing with NumPy to web development with Flask and Django, these libraries offer powerful tools that can help developers build efficient and scalable solutions. Mastering these libraries not only boosts productivity but also deepens understanding of Python’s capabilities.
FAQs
1. What is the difference between NumPy and Pandas?
NumPy is primarily used for numerical operations and array manipulations, while Pandas is designed for data manipulation and analysis using DataFrames and Series. Pandas builds on top of NumPy and provides higher-level data structures.
2. When should I use Matplotlib versus Seaborn?
Matplotlib is a versatile plotting library that offers extensive customization options for various plot types. Seaborn, built on top of Matplotlib, provides a more user-friendly interface for statistical plots and integrates seamlessly with Pandas DataFrames. Use Seaborn for quick and aesthetically pleasing statistical plots, and Matplotlib for detailed customization.
3. Is Flask or Django better for web development?
Flask is lightweight and flexible, suitable for smaller applications or when you need more control over the components of your app. Django is a more feature-rich framework with built-in tools for larger applications, including an ORM and an admin interface. Choose Flask for simplicity and Django for a comprehensive solution.
4. How does Scikit-learn support machine learning?
Scikit-learn provides a variety of machine learning algorithms and tools for tasks such as classification, regression, clustering, and model evaluation. It includes features for data preprocessing, model selection, and cross-validation, making it a robust tool for building and evaluating machine learning models.
5. Can I use these libraries together in a project?
Yes, these libraries are often used together in projects. For example, you might use Pandas for data manipulation, NumPy for numerical operations, Matplotlib or Seaborn for data visualization, and Scikit-learn for machine learning tasks. Flask or Django can be used to develop web applications that integrate these data processing and analysis tools.