Creating responsive designs in Flutter ensures that your app looks great on any device, regardless of screen size or orientation. This guide will walk you through the essential steps to achieve responsive designs using Flutter’s powerful tools and widgets.
Step 1: Understanding Screen Sizes and Breakpoints
Before diving into the implementation, it’s crucial to understand the different screen sizes and breakpoints. Common breakpoints include:
- Mobile (small screens): <600dp
- Tablet (medium screens): 600dp – 1200dp
- Desktop (large screens): >1200dp
Tip: Use MediaQuery
to get the size of the current screen and adjust your layout accordingly.
Step 2: Using Flexible and Expanded Widgets
The Flexible
and Expanded
widgets are essential for creating flexible layouts. These widgets help distribute space within a Row
or Column
.
Trick: Use Expanded
to make a widget fill the available space, and Flexible
to allow a widget to resize within its constraints.
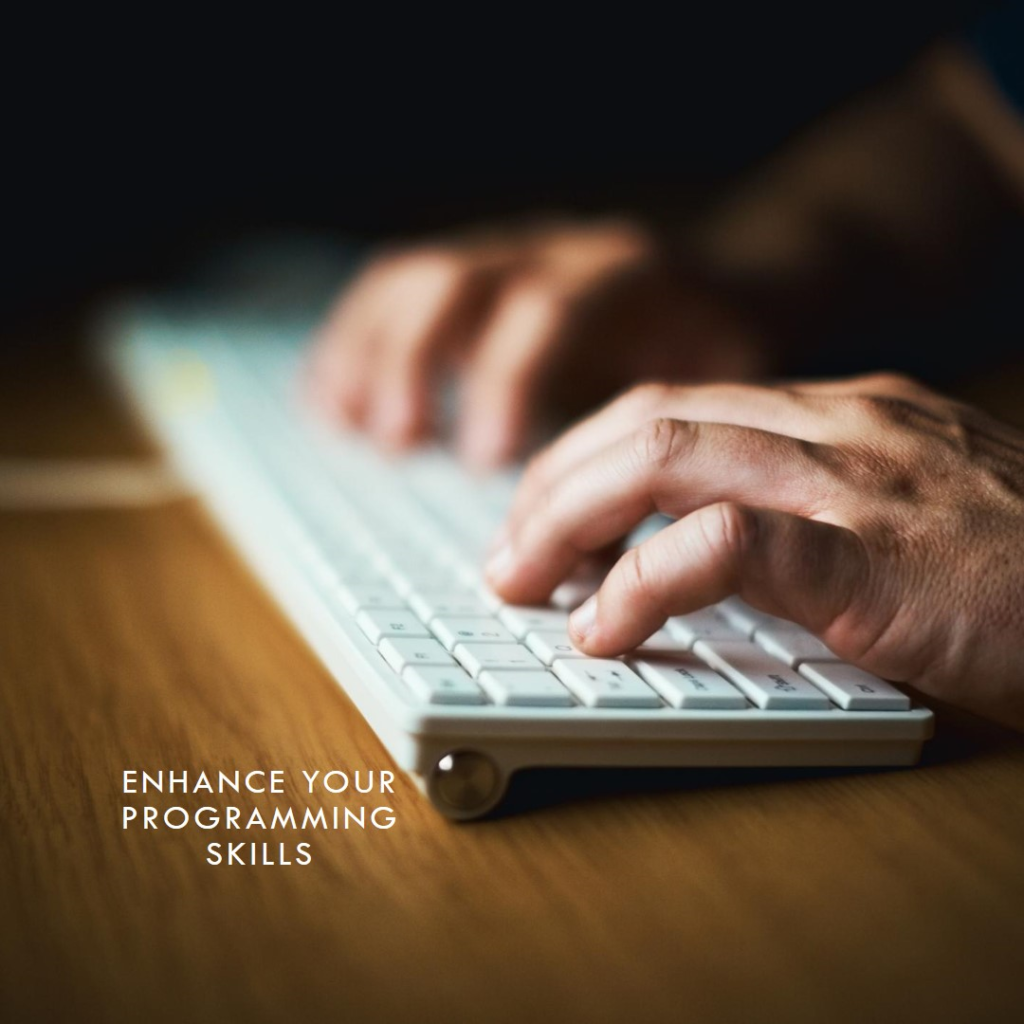
Step 3: Leveraging LayoutBuilder
The LayoutBuilder
widget helps you build responsive layouts by providing the parent widget’s constraints.
LayoutBuilder(
builder: (context, constraints) {
if (constraints.maxWidth < 600) {
return MobileLayout();
} else if (constraints.maxWidth < 1200) {
return TabletLayout();
} else {
return DesktopLayout();
}
},
);
Step 4: Using MediaQuery
MediaQuery
provides information about the size and orientation of the current screen. Use it to create adaptive layouts.
var screenWidth = MediaQuery.of(context).size.width;
var screenHeight = MediaQuery.of(context).size.height;
Tip: Adjust padding, margins, and font sizes based on the screen dimensions obtained from MediaQuery
.
Step 5: Implementing Responsive Images and Icons
Ensure images and icons scale properly on different devices. Use BoxFit
for images and Icon
size properties for icons.
Image.asset(
'assets/image.png',
width: screenWidth * 0.5,
fit: BoxFit.cover,
);
Step 6: Using Responsive Packages
Flutter offers several packages to aid in creating responsive designs, such as flutter_screenutil
and responsive_builder
.
Trick: These packages simplify the process of managing different screen sizes and orientations, allowing you to focus on the design.
FAQs
Q1: How can I make my Flutter app responsive to different screen sizes?
A1: Use MediaQuery
, LayoutBuilder
, Flexible
, and Expanded
widgets to adjust your layout based on screen size.
Q2: What is the best way to handle different orientations in Flutter?
A2: Use OrientationBuilder
to detect changes in orientation and adjust your layout accordingly.
Q3: How do I scale text in Flutter for different screen sizes?
A3: Use the flutter_screenutil
package or MediaQuery
to adjust text size based on screen dimensions.
Q4: Can I create responsive layouts without using third-party packages?
A4: Yes, Flutter provides built-in widgets like MediaQuery
, LayoutBuilder
, and Flexible
that can be used to create responsive layouts.
Q5: How do I test my Flutter app on different screen sizes and orientations?
A5: Use Flutter’s built-in emulator configurations and responsive testing tools in your IDE to simulate different screen sizes and orientations.
Conclusion
Creating responsive designs in Flutter ensures that your app delivers a consistent and polished experience across all devices. By understanding screen sizes and breakpoints, leveraging widgets like Flexible
, Expanded
, LayoutBuilder
, and MediaQuery
, and utilizing responsive packages, you can build adaptable and beautiful UIs. With these steps and tips, you’ll be well-equipped to tackle any responsive design challenge in Flutter. Happy designing!