Design patterns are proven solutions to common problems in software design. They provide a standard terminology and are specific to particular scenarios. In this blog post, we’ll explore some of the most important design patterns in Java, including Singleton, Factory, and others, and discuss how they can be applied to improve your software architecture.
What are Design Patterns?
Design patterns are typical solutions to common problems in software design. They are like blueprints that you can customize to solve a particular design problem in your code. They were popularized by the “Gang of Four” book, Design Patterns: Elements of Reusable Object-Oriented Software.
The Singleton Pattern
The Singleton pattern ensures that a class has only one instance and provides a global point of access to it. This pattern is useful for managing shared resources like database connections, configuration settings, or logging.
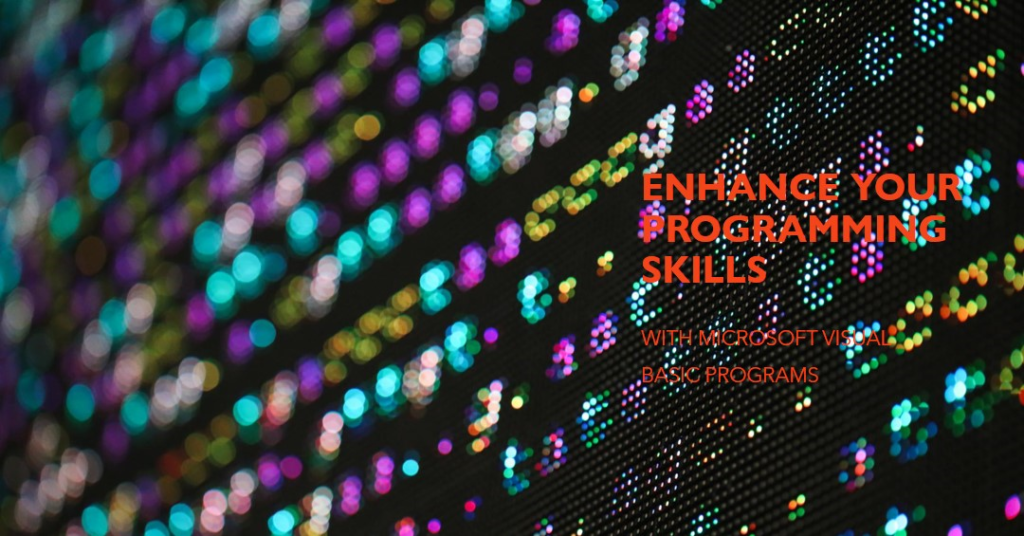
Here’s an example of the Singleton pattern in Java:
public class Singleton {
private static Singleton instance;
private Singleton() {}
public static Singleton getInstance() {
if (instance == null) {
instance = new Singleton();
}
return instance;
}
}
In this example, the getInstance()
method ensures that only one instance of the Singleton
class is created.
The Factory Pattern
The Factory pattern is a creational pattern that provides an interface for creating objects in a superclass, but allows subclasses to alter the type of objects that will be created. This pattern is useful for managing and maintaining the creation process of objects.
Here’s an example of the Factory pattern in Java:
interface Shape {
void draw();
}
class Circle implements Shape {
public void draw() {
System.out.println("Drawing a Circle");
}
}
class Square implements Shape {
public void draw() {
System.out.println("Drawing a Square");
}
}
class ShapeFactory {
public Shape getShape(String shapeType) {
if (shapeType == null) {
return null;
}
if (shapeType.equalsIgnoreCase("CIRCLE")) {
return new Circle();
} else if (shapeType.equalsIgnoreCase("SQUARE")) {
return new Square();
}
return null;
}
}
In this example, the ShapeFactory
class is used to create instances of different shapes based on the input type.
Other Important Design Patterns
- Observer Pattern: Defines a one-to-many dependency between objects so that when one object changes state, all its dependents are notified and updated automatically.
import java.util.ArrayList; import java.util.List; class Subject { private List<Observer> observers = new ArrayList<>();public void attach(Observer observer) { observers.add(observer); } public void notifyAllObservers() { for (Observer observer : observers) { observer.update(); } }} interface Observer { void update(); } class ConcreteObserver implements Observer { public void update() { System.out.println("State changed!"); } }
- Decorator Pattern: Allows behavior to be added to individual objects, dynamically, without affecting the behavior of other objects from the same class.
interface Coffee { String getDescription(); double cost(); } class SimpleCoffee implements Coffee { public String getDescription() { return "Simple coffee"; }public double cost() { return 2.0; }} class MilkDecorator implements Coffee { protected Coffee coffee;public MilkDecorator(Coffee coffee) { this.coffee = coffee; } public String getDescription() { return coffee.getDescription() + ", milk"; } public double cost() { return coffee.cost() + 0.5; }}
- Strategy Pattern: Defines a family of algorithms, encapsulates each one, and makes them interchangeable. This pattern lets the algorithm vary independently from the clients that use it.
interface PaymentStrategy { void pay(int amount); } class CreditCardPayment implements PaymentStrategy { public void pay(int amount) { System.out.println("Paid " + amount + " using Credit Card."); } } class PayPalPayment implements PaymentStrategy { public void pay(int amount) { System.out.println("Paid " + amount + " using PayPal."); } } class ShoppingCart { private PaymentStrategy paymentStrategy;public void setPaymentStrategy(PaymentStrategy paymentStrategy) { this.paymentStrategy = paymentStrategy; } public void checkout(int amount) { paymentStrategy.pay(amount); }}
Conclusion
Understanding and applying design patterns can significantly enhance your Java programming skills by providing tried and tested solutions to common design problems. Patterns like Singleton and Factory simplify object creation, while others like Observer and Strategy provide flexible and reusable design solutions. By integrating these patterns into your projects, you can create more robust, maintainable, and scalable software.
If you found this exploration of design patterns helpful, be sure to check out our other articles on advanced design patterns and best practices in software architecture. Stay tuned for more insights and tips to improve your Java programming and design skills.